Orlando, Florida is a city that is known for its world-renowned theme parks and attractions that are perfect for families with children. From thrilling rides to educational exhibits, there is something for everyone to enjoy in Orlando.
One of the main attractions in Orlando is Walt Disney World. This massive theme park complex features four separate parks: Magic Kingdom, Epcot, Hollywood Studios and Animal Kingdom. Each park offers a unique experience, with Magic Kingdom offering classic Disney rides and characters, Epcot offering a cultural experience, Hollywood Studios featuring some of the most popular attractions and Animal Kingdom is full of live animals and nature-inspired attractions.
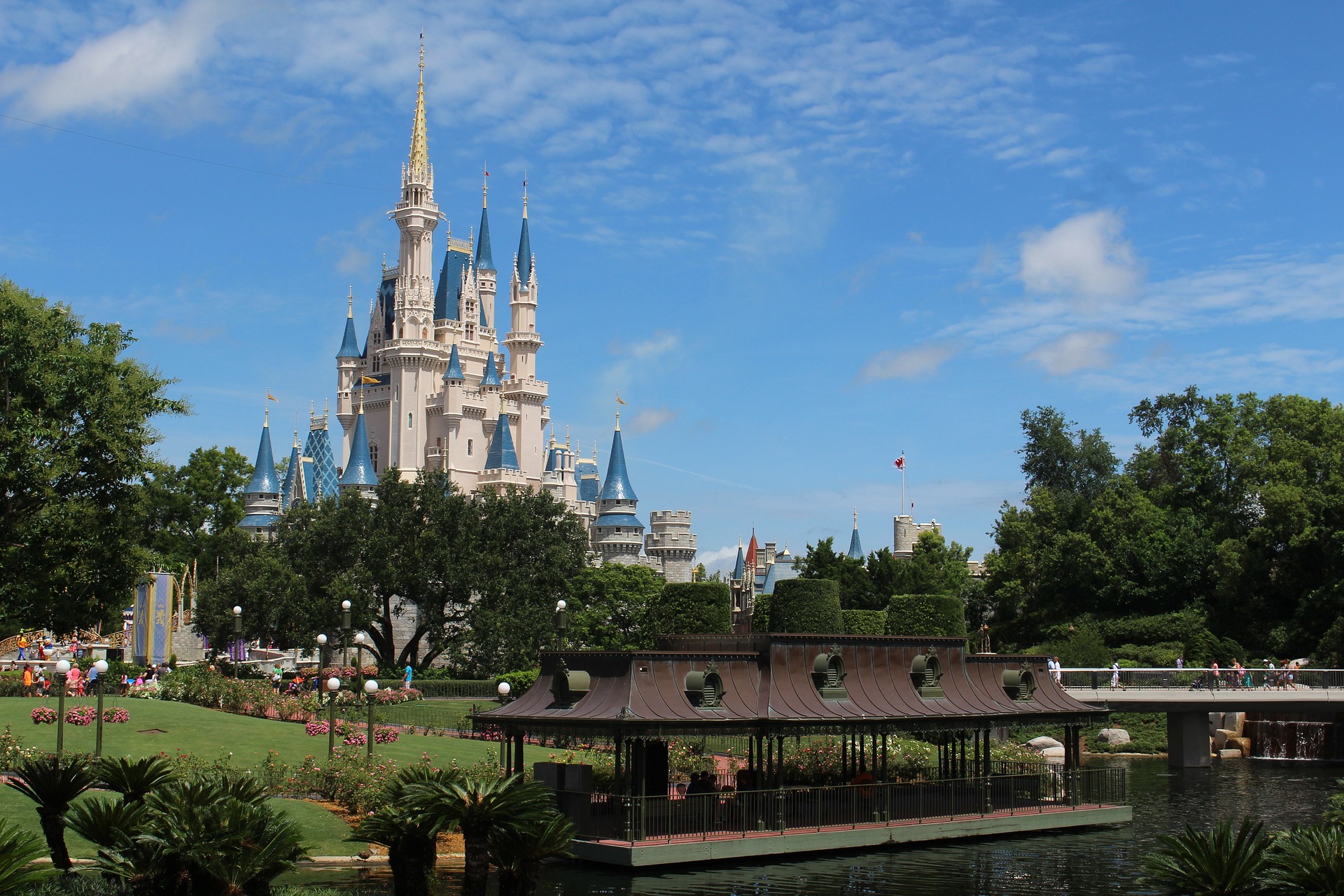
Another popular attraction in Orlando is Universal Studios.
This park features several exciting rides and shows based on popular movies and TV shows, including Harry Potter and the Forbidden Journey, Revenge of the Mummy and The Simpsons Ride.
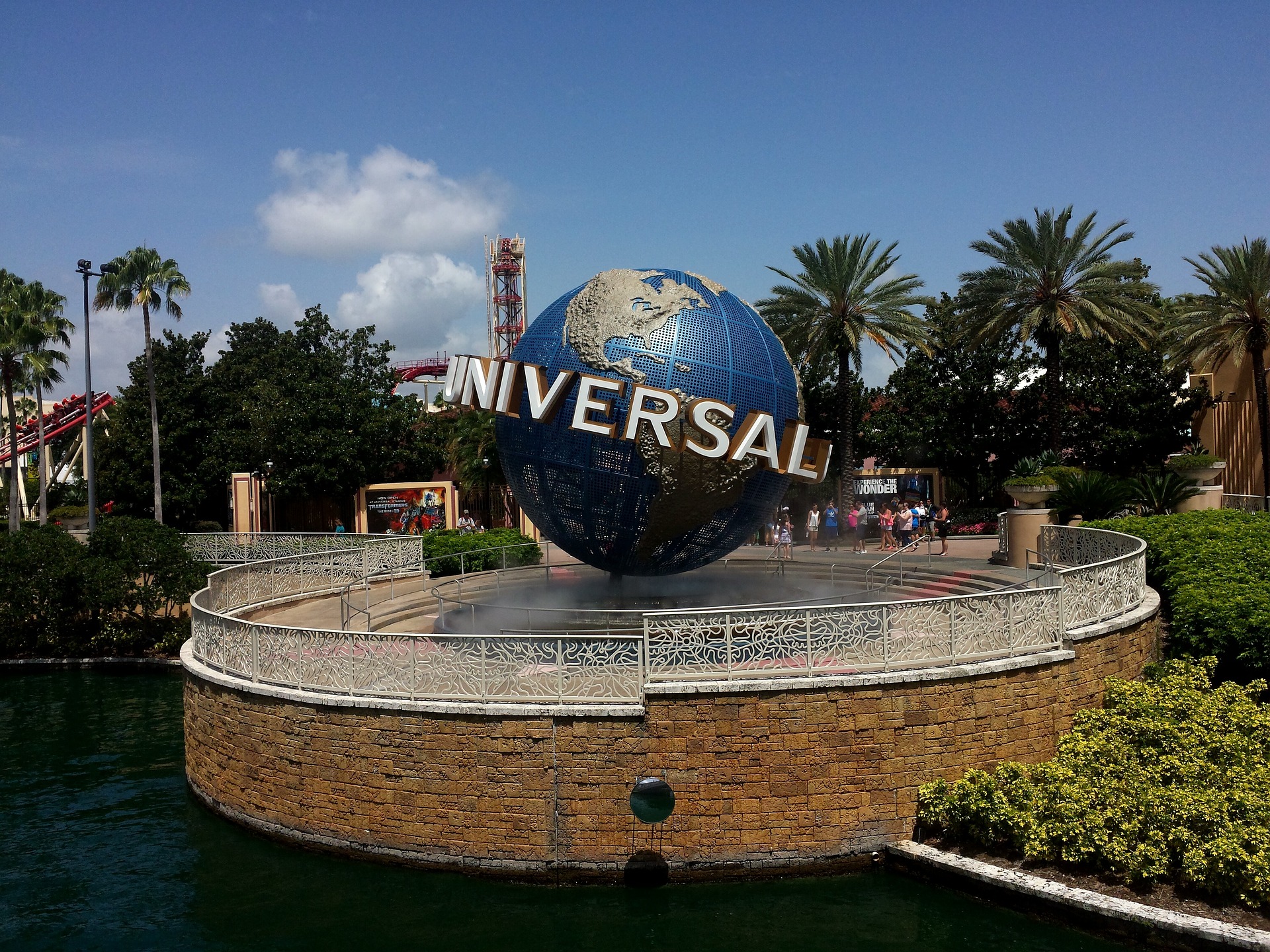
SeaWorld Orlando is another family-friendly destination in the city. This marine-life theme park offers a variety of shows and exhibits, as well as rides such as the Kraken Unleashed roller coaster and the Journey to Atlantis water ride.
The park also features an array of marine animals, including dolphins, sea lions, and sharks.
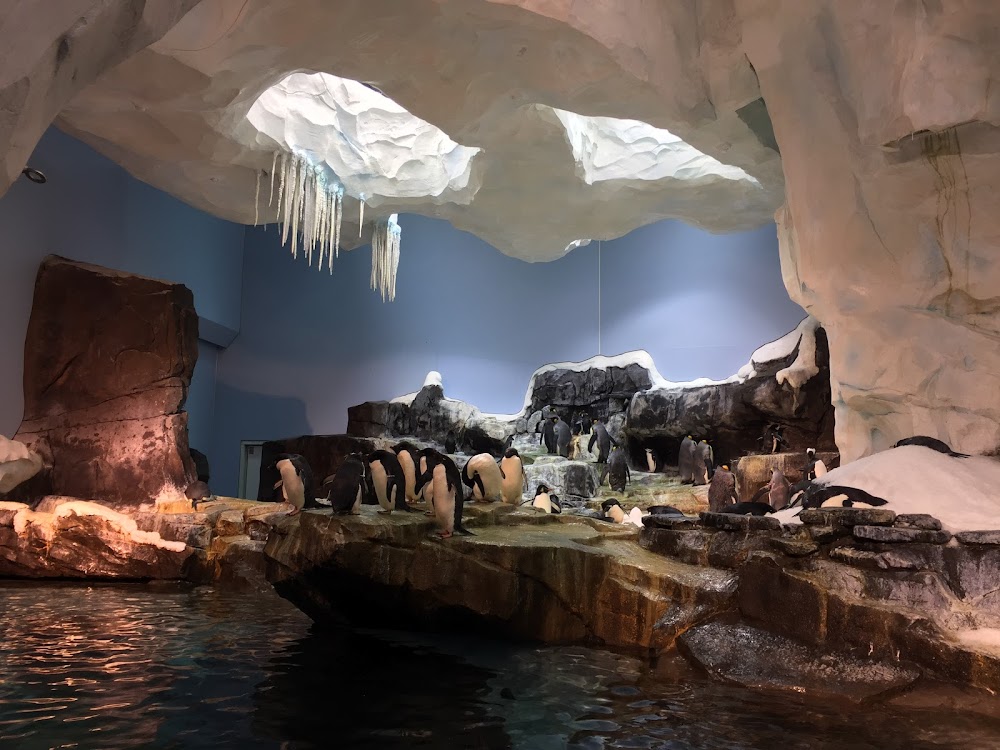
For families looking for an educational experience, Orlando offers a variety of museums and science centers. The Orlando Science Center is a hands-on museum that offers interactive exhibits, live demonstrations, and a planetarium.
The Florida Museum of Natural History is another great option, featuring a variety of exhibits on Florida’s natural history, as well as a butterfly garden.
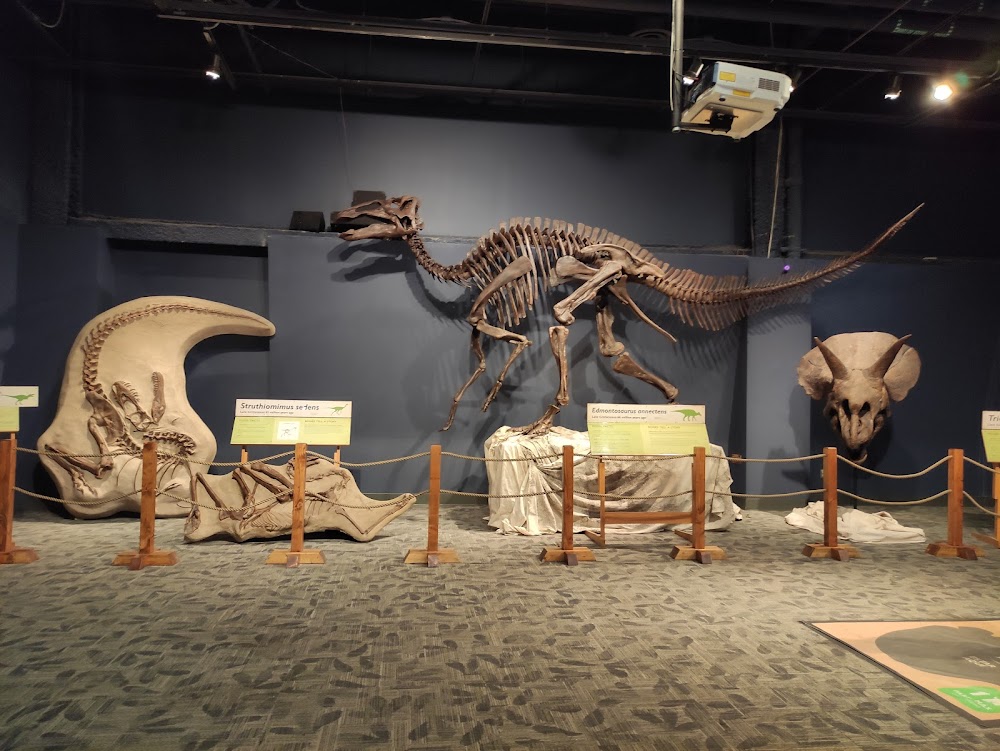
In addition to the theme parks and attractions, Orlando offers a variety of other activities that are perfect for families with children. The city is home to a number of water parks, such as Aquatica, Typhoon Lagoon and Blizzard Beach, which offer a fun way to cool off on a hot day. Additionally, there are many shopping and dining options in the city, as well as a variety of outdoor recreational activities, such as golfing, fishing and boating.
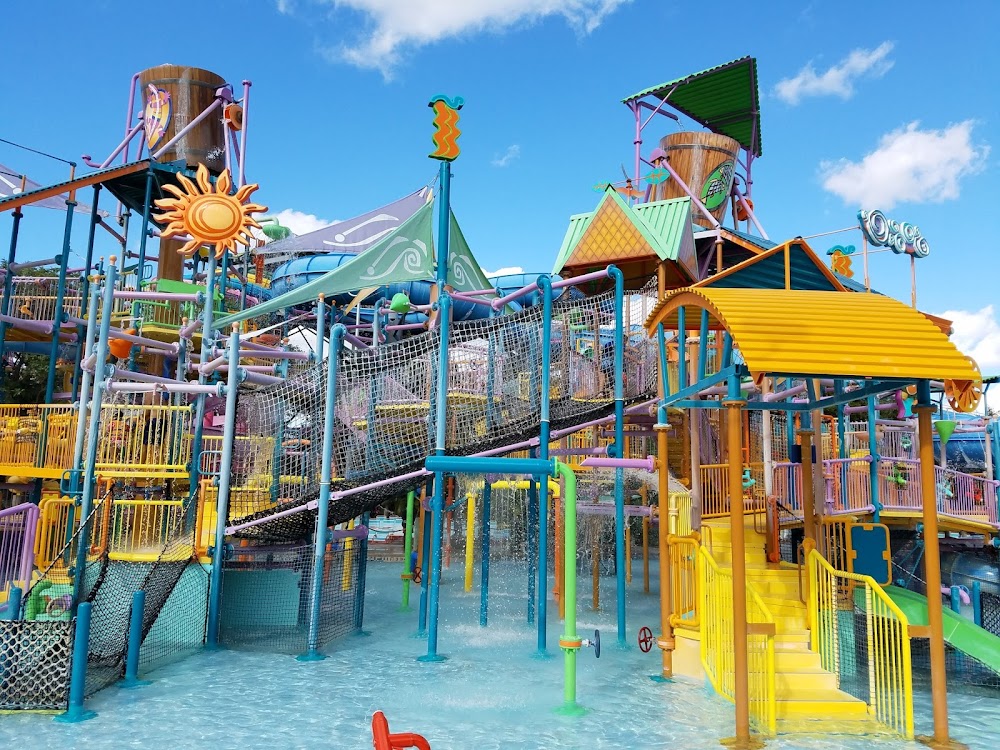
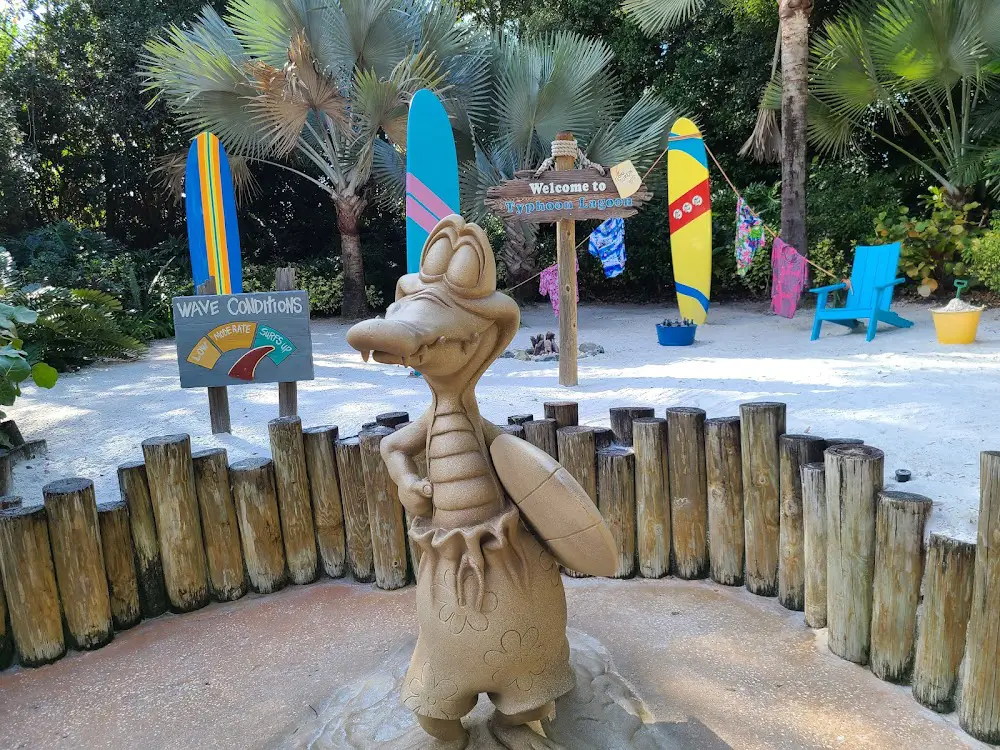
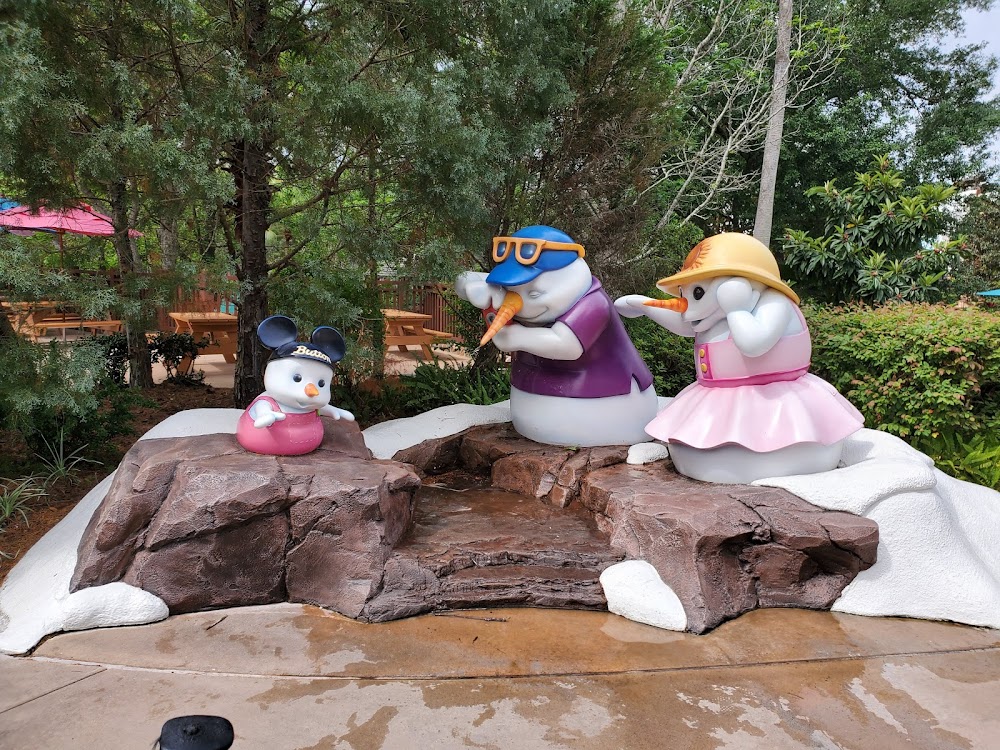
Overall, Orlando is a city that offers something for everyone, making it a perfect destination for families with children. With its world-renowned theme parks, educational museums, and variety of other activities, there is no shortage of things to do and see in Orlando.